Write a function that given an expression tree with the following properties:
- leaves are represented with * and internal node with +.
- each node has either 0 or 2 children.
Given the preorder traversal of a tree, construct the tree.
Example: for the following preorder ++*+*** , the tree should look like:
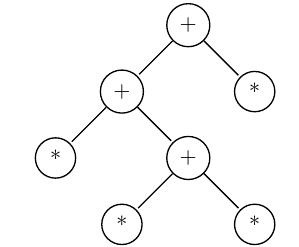
Difficulty level

This exercise is mostly suitable for students
Btree buildFromPreorder_h(char A[], int *counter)
{
Btree node;
if(A)
{
node=(Btree)malloc(sizeof(struct node));
node->data=A[*counter];
node->left=node->right=NULL;
if(A[*counter]=='*')
return node;
(*counter)++;
node->left = buildFromPreorder_h(A,counter);
(*counter)++;
node->right = buildFromPreorder_h(A,counter);
return node;
}
return NULL;
}
Btree buildFromPreorder(char A[])
{
int counter = 0;
return buildFromPreorder_h(A, &counter);
}
Back to the list of exercises
Looking for a more challenging exercise, try this one !!
